Using typed hooks
If you import the Easy Peasy hooks directly you need to provide your model to them so that your stores typing is available within the mapping functions.
import { useStoreState } from 'easy-peasy';
import { StoreModel } from '../model'; // π you would need to do this
function MyComponent() {
// and then this π
const foo = useStoreState<StoreModel>(state => state.foo);
}
That is slightly cumbersome, therefore for convenience sake, we expose a createTypedHooks API which allows you to create versions of the hooks which are pre-bound within your StoreModel. Using the hooks returned by this API allows you to avoid having to provide your StoreModel every time you use one of the hooks.
We therefore recommend that you use the createTypedHooks API to create typed hooks and then export them so that you can use them within your components.
Exporting the typed hooks
The following example will demonstrate how we can create and export our typed hooks.
// src/hooks.ts
import { createTypedHooks } from 'easy-peasy'; // πimport the helper
import { StoreModel } from './model'; // π import our model type
// Provide our model to the helper π
const typedHooks = createTypedHooks<StoreModel>();
// π export the typed hooks
export const useStoreActions = typedHooks.useStoreActions;
export const useStoreDispatch = typedHooks.useStoreDispatch;
export const useStoreState = typedHooks.useStoreState;
Using the typed hooks
We can now import the typed hooks into a component.
import { useStoreState } from '../hooks'; // π import the typed hook
function TodoList() {
const todos = useStoreState(state => state.todos.items);
return (
<ul>
{todos.map(todo => <li>{todo}</li>)}
</ul>
);
}
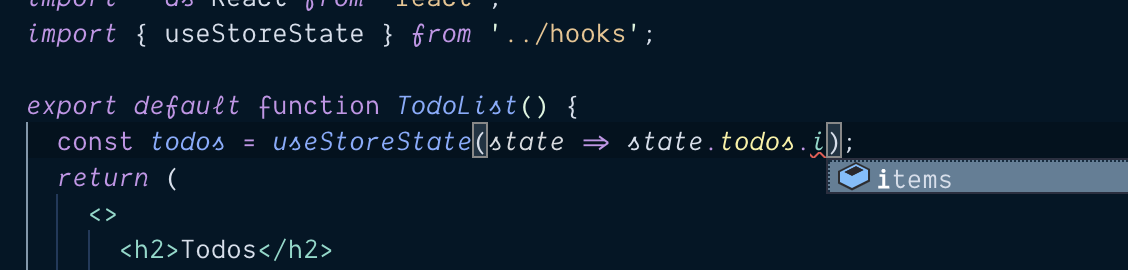
Review
You can view the progress of our demo application here.